Building notification service for doorbell [Project]
/ 9 min read
Introduction
Let me tell you a story. Shortly after the COVID peak, I was still working from home. My siblings were also staying at home. One thing was common between all of us, and that was we were all plugged into a computer or a tablet. I would usually be listening to music, or podcast while working while my siblings were either studying, watching a movie, sports or just live streams. A problem that came with it was our collective deafness to outside noise and alerts. Someone could literally break into the house and we would not notice until it was too late. Often times, someone would be at our door and we would just not hear the doorbell ring. So, I wondered how convenient it would be, if we could receive a notification on our devices whenever someone rang the doorbell.
Now, I get what you’re thinking, “Why not buy an off the shelf Ring1 doorbell? It does all the things you need.” And my response to that would be “Meh, it’s not interesting”. I could have used that but it’s such a costly device. The cost does not justify the solution employed for the problem. It’s also interesting and fills me with joy to build something yourself that solves your problem.
Premise
The initial thoughts that were running through my mind were to use some sort of device that could detect the bell ring and ping an app on my device which was listening on some port. The only device I could think of was a Raspberry Pi watching for signal changes on one of it’s GPIO pins and send a request to a simple python server listening on my Mac. This app would then show a notification.
Initial Problems
Now, the idea looks pretty simple on paper but I had a few more things to figure out. I had never owned a Raspberry PI before and after COVID its supply was also limited. The app that I was planning on making to show the notifications would only work for my Mac. I wasn’t too keen on making a dedicated native app for a Windows machine, an iPad, Android phones etc. For Mac and PC, a simple script would work but then I would have to atleast write a basic app for phone and iPad.
Brainstorming and Proof Of Concept
After scratching my head quite a bit, I thought, can I make use of browser push notifications? I always considered browser notifications annoying because some random website would always ask you to subscribe and if you did, they’ll spam you all the time. That’s all I knew about browser notifications. So, before I start buying PI and other stuff, I thought of at least having a proof of concept and validate if this idea of mine would actually work.
I began googling about how to generate browser notifications. It has been quite a long time since I used HTML & Javascript. I had done a deep dive on it during school days, a few times during college and then left it after losing interest because I was unable to keep up with ever emerging JS frameworks. Anyway, it was time to delve into JS and use it to generate notifications. I wrote a bunch of JS code using browser APIs and was finally able to make it work on my local machine2.
More Problems
After the POC, when I tried to subscribe to the notifications on a different device, I was unable to. I would get cryptic error and due to my lack of experience, it just did not make sense. Again after a bit of googling, I found out it requires an HTTPS website to make it work. Me testing the subscription functionality earlier locally would work because it was hosted on localhost
which is trusted. I was hit with yet another roadblock. I began looking for other options. Should I create a self-signed certificate and install that on all the devices? Would that even work? I had no idea.
I began searching for free website hosting services with SSL certificate. It finally summed up to me using Firebase to host a free HTTPS website. Now, the APIs for web push notifications were a little different here but it did not bother me. I just churned down the examples and came out with another working solution. Now, I could open the website on any browser on any device and I was able to subscribe to the notifications.
How Web Push Notification work with Firebase?
This is just a quick info on how it works. I’m not deep diving into the nitty gritty.
I setup a Firebase website that has free hosting. It was just a basic HTML website using bootstrap to prettify it (cause that’s the only thing I had used before besides pure CSS). I added a button for user to subscribe. It internally called a Firebase API to generate an authorization key which will be the used to send notification. Anyone with this key can send notification to user by sending a POST
request to the fcm.googleapis.com/fcm/send
with the key set in the authorization
header. The website needs to be an SSL certified website so that the source of the notification can be verified.
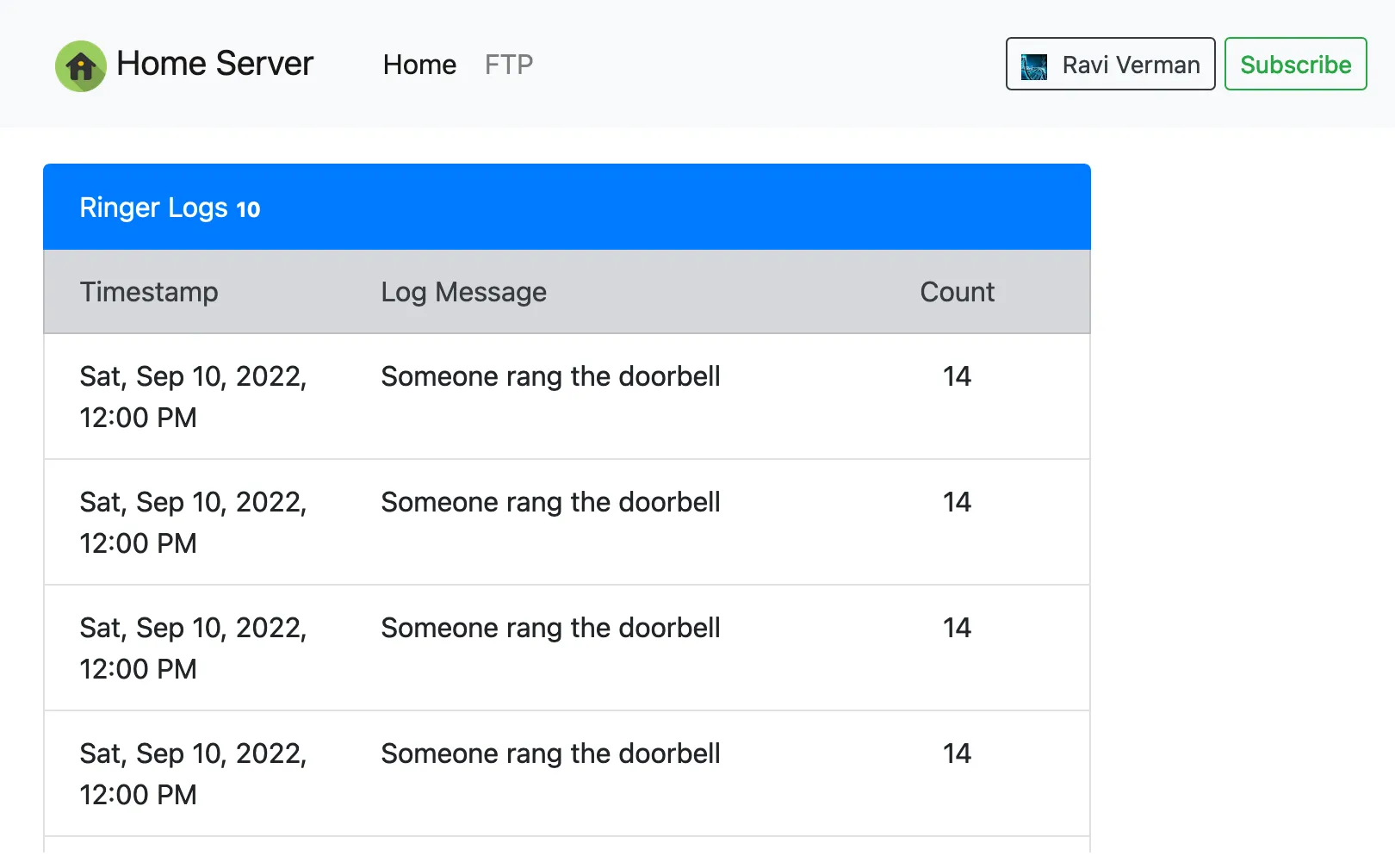
I later added user authentication to let only logged in user to subscribed to the notifications. A user can still stumble on by website, login, subscribe and get the notifications. I added an extra layer to avoid this. More on this later.
Re-iteration
Just to summarize the things above, this is how it will work. Any user can go this website hosted on Firebase, authenticate, and then subscribe to the notifications. The Raspberry PI will fetch the notification info for all users and when it detects the ring on it’s GPIO pin and it will send the notifications to all the subscribed users. It will also keep logs of the past notifications and display it on the website.
The setup would look something like this:
+------+ -> GPIO +------+ |SWITCH|--------------------------------+ PI | +------+ PIN | | RING +------+ || || +------+ SUBSCRIBE-> +--------+ INTERNET || | USER |=============|FIREBASE|============++ +------+ <-NOTIFY +--------+ <->
Now, that I have the proof of concept and know that it’ll work, I can now actually spend money to buy stuff.
Google helps out
After sleeping on this whole idea, I was having second thoughts about using a Raspberry PI, a general purpose computer, to do one small little thing. It is capable of running loads of things but that’s the only device I knew had GPIO pins and an onboard WiFi chip and can send request to Google servers to send notifications.
I probably had searched a lot about PI and stuff on Google, and one day I see a recommendation on my YouTube feed. It was a micro-controller with an onboard WiFi. This was perfect and exactly what I needed. A small board, cheap, and more than enough compute for the purpose. It had GPIO pins, 4MB of flash memory with whole TCP stack 🤯. It was ESP82663 and went on full deep down researching about it.
Building the idea
So, I starting buying stuff and I bought the following items
- ESP8266 micro-controller
- A multi meter (because I don’t want to blow things up)
- A breadboard and jumpers
- A Raspberry PI ( yep, still bought it )
- A pair of 220 AC to 5/3.3V DC transformers
I still bought a Raspberry PI because I can do so many other stuff with it. It will act as a middle ware to receive request from the ESP8266 and send notifications. I was earlier planning on using an old laptop for this purpose.
Programming the ESP8266
I learned how to use this micro-controller and wrote code that
- Connected to WiFi at startup.
- Detected voltage change on one of its GPIO pins, sends out a
POST
request to Raspberry PI.
Extra stuff that I added eventually
- A web server on ESP8266 with an endpoint
/status
: To check the status of the micro-controller./update
: That puts it into flash mode and I can update the firmware over the WiFi. This device would be mounted high on the wall with the bell./testbell
: That can send a test request to the PI server to trigger notifications. During development, I don’t want to go out and ring the bell to test if this whole thing works./restart
: To restart the device.
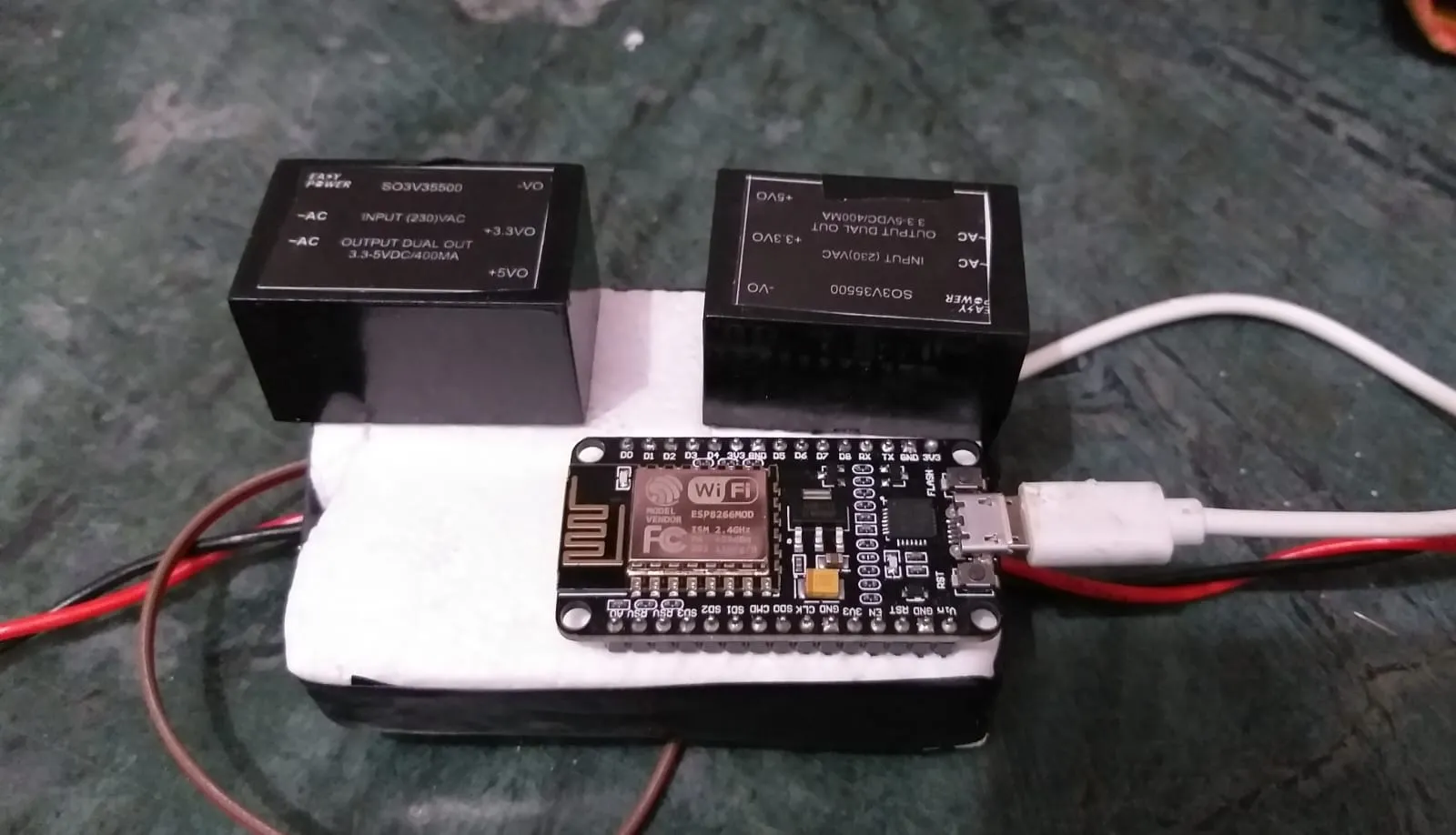
Programming the Raspberry PI
This Raspberry PI is running a web server to handle requests from the micro-controller. It’s also listening for any updates from Firebase for any new subscribers. The user notification authorization key is fetched and stored locally. When the micro-controller send the ring request, the notifications are forwarded to the users with a message along with the time the bell was rung.
When the micro-controller sends a test request (using /testring
), it sends the request to PI with a different request code, it results in notifications being sent to only my device.
Additionally, it also maintained an access list locally to only send notifications to certain approved users. This was because any authenticated user can subscribe but I don’t want to send them notification if they’re not on the approved list.
Little orthogonal, but the PI now runs other things as well like Pi-Hole, a DNS based ad-blocking service, that blocks ads for all devices connected to my home WiFi. It’s also an entry point to my home network that I can connect to through a VPN and resolve issues remotely.
Final Look
The new design looks as follows:
+------+ -> +------+ -> +------+ |SWITCH|---------| ESP |---------------| PI | +------+ GPIO| 8266 | | | RING +------+ +------+ || || +------+ SUBSCRIBE-> +--------+ INTERNET || | USER |=============|FIREBASE|============++ +------+ <-NOTIFY +--------+ <->
If you’ve not already noticed, this notification mechanism which was originally planned to work for local network would now work over the internet. If anyone rings a bell at my home, I would receive a notification, no matter where I was.
This project began with just an idea. I had no prior knowledge on working with Raspberry PI or ESP8266 micro-controller. I had also never worked on Firebase before. But during the course of this project, I not only learned these things but also enjoyed it all throughout.